Overview
ImpEx decorators and translators provide a way to add custom logic and modifications to the ImpEx process without modifying the core code of the platform. These features contribute to the flexibility and extensibility of the SAP Commerce Cloud solution when dealing with data import and export tasks.
​
The Impex decorator and translator are used by Hybris in the Impex life cycle to change data before it is processed (imported or exported).
​
There are basically two differences between them :
-
Impex’s life cycle : Decorator and translator are being executed in different instant of the Impex life cycle.
-
Impex Inputs/Output : Decorator and translator have different type of input and output.
​
​
Translator
​
An ImpEx translator is a concept within SAP Hybris Commerce that facilitates data transformation and mapping during import and export operations. ImpEx translators play a crucial role in managing and manipulating data as it is moved into or out of the Hybris platform.
​
Data integration often requires data transformation or mapping because source data may have different formats, structures, or codes than what is expected in the SAP Commerce Cloud system. ImpEx translators are used to bridge these gaps by transforming and mapping data during the import and export process.
​
​
Translator Life Cycle: Translator life cycle has manly two steps :
​
Step 1: The impex will be executed and row will be transformed in to the item.
Step 2: The transformed item is now processed with translator changes.
​
​
​
​
​
​
​
​
Practical Use Case:
You are required to create a password encoder translator that will translate or encode the user password. In this case, you might have a translator within your ImpEx script that converts the password from the source format to the encoded format.
INSERT_UPDATE Unit; uid[unique=true]; password[translator=com.hybris.impex.EncodedPasswordTranslator]
;user1; password1 ;
user2; password2
​
In the example above, [translator=com.hybris.impex.EncodedPasswordTranslator] represents the translator responsible for converting the password.
​
​
Custom Translator Implementation Steps:
To implement a custom translator in SAP Hybris Commerce, you need to create a custom Java class that performs the required data transformation or mapping logic and then configure your ImpEx script to use this custom translator. Here are the steps to implement a custom translator:
​
Step 1 (Create a Custom Translator Class) : In your SAP Commerce project, create a custom Java class that will serve as your translator. This class should contain a method that performs the data transformation logic. You have to extend the AbstractValueTranslator class and give the implementation within importValue and exportValue methods.
Here's an example:​​​
package com.hybris.impex;
import de.hybris.platform.impex.jalo.translators.AbstractValueTranslator;
import de.hybris.platform.jalo.Item;
import de.hybris.platform.jalo.JaloInvalidParameterException;
import de.hybris.platform.jalo.user.User;
public class EncodedPasswordTranslator extends AbstractValueTranslator
{
@Override public Object importValue(String cellValue, Item item) throws JaloInvalidParameterException
{
final User user = (User) item;
final String passwordEncoded = encodePassword(cellValue);
((User) item).setPassword(passwordEncoded);
return item;
}
@Override public String exportValue(Object item) throws JaloInvalidParameterException
{
// don't export users password :p return "*********";
}
}
​​
​
Step 2 (Configure the Custom Translator in Spring Configuration) : In your project's Spring configuration (usually in an XML file), you need to define a bean for your custom translator class. This step tells the SAP Commerce platform where to find and load your custom translator. Here's an example of how to configure it:
<bean id="encodedPasswordTranslator" class="com.hybris.impex.EncodedPasswordTranslator" />
​
​
Step 3 (Use the Custom Translator in an ImpEx Script) : In your ImpEx script, you can use your custom translator by specifying it within square brackets in the relevant ImpEx command, such as INSERT, UPDATE, or REMOVE. Provide the necessary input data to the custom translator method. Here's an example:
​
INSERT_UPDATE Unit; uid[unique=true]; password[translator="encodedPasswordTranslator"]
;user1; translatortespassword1 ;
;user1; translatortespassword2 ;
​
​
​
​
​
Decorator
​
Impex decorators are used to add custom logic to the Impex process. They intercept Impex statements and provide an opportunity to modify data, perform to execute additional actions.​
Decorator life cycle: Decorator execution happens in early stage of impex processing. The process is divided in the following two steps:
Step 1: The decorator process the impex and creates a edited version of the impex.
Step2: The processed impex in the previous part is executed and converted to the Jalo Objects.
Practical Use Case: We want to combine the price value and price sign from a row of impex and combine them to generate a new column value by combining both.
Example : We have following an example of unprocessed and decoareated impex sample.
Initial Impex:
INSERT_UPDATE Price; code[unique=true]; currency; priceValue[cellDecorator=com.custom.hybris.impex.PriceCurrencyValueDecorator, curr_index=2];
;price_sample; €; 110
;price_sample2; €; 98
;price_sample3; $; 89
​
Decorated Impex :
INSERT_UPDATE Price; code[unique=true]; currency; priceValue[cellDecorator=com.custom.hybris.impex.PriceCurrencyValueDecorator, curr_index=2];
;price_sample; €; 110€
;price_sample2; €; 98€
;price_sample3; $; 89$
​
​
Custom Decorator Implementation: We will create a decorator that will create a decorated price and currency value discusses above.
​
package com.custom.hybris.impex;
import de.hybris.platform.impex.jalo.header.AbstractColumnDescriptor;
import de.hybris.platform.impex.jalo.header.AbstractImpExCSVCellDecorator;
import de.hybris.platform.impex.jalo.header.HeaderValidationException;
import java.util.Map;
public class PriceCurrencyValueDecorator extends AbstractImpExCSVCellDecorator
{
private String currencyIndex;
@Override public void init(AbstractColumnDescriptor column) throws HeaderValidationException
{
super.init(column);
// retrieve currency index
index currencyIndex = column.getDescriptorData().getModifier("curr_index");
}
@Override public String decorate(int index, Map<Integer, String> map)
{
// get currency value
final String currency = map.get(currencyIndex);
// get current cell value
(priceValue) final String priceValue = map.get(index);
// get output : priceValue + currency
return priceValue + currency;
}
}
​
We can use the decorator in impex, by using the cellDecorator attribute to config your PriceCurrencyValueDecorator and currency_index to refer to the index of the cell currency in the impex.
​
INSERT_UPDATE Price; code[unique=true]; currency; priceValue[cellDecorator=com.custom.hybris.impex.PriceCurrencyValueDecorator, curr_index=2];
;price_sample; €; 110
;price_sample2; €; 98
;price_sample3; $; 89
​
​
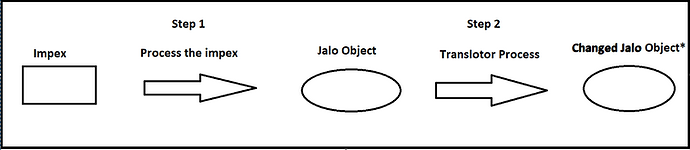
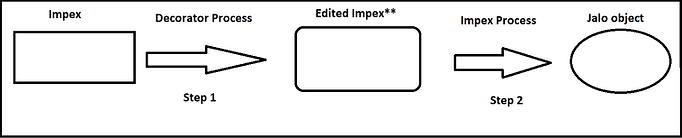