Overview
​
Business processes are workflows that describe how various operations and transactions are conducted within an e-commerce environment. These processes are crucial for the efficient operation of an e-commerce platform.
​
On Cluster aware processing, the Business Process Feature is built. Accordingly, the business process can be distributed throughout the nodes of a Hybris cluster and operate as needed. This suggests that the process is carried out in an asynchronous fashion. The user who wants to send an email or place an order can therefore see every business transaction in clear view.
The process flow is technically described in an XML file, and each stage requires the implementation of a bean so that business logic may be executed inside of each state and produce an output that directs the process to a particular new state. One must be aware that a Transition is what moves the process from one state to another.
The fact that the state is constantly persistent is a significant advantage of the business process feature. As a result, in the event of a system crash, the processengine will remember its previous location and resume as soon as the processengine context is restored. With it, there is a significant guarantee that, with the exception of a statistically small number of cases, the process will run safely and won't repeat any steps.
​
Business Process Engine
Business process engine defines the business process through XML and run this process in the asynchronous way. The XML file contains each step and conditions in the ordered way. We can see these processes are ran in the order of definitions.
We can defined the waiting events, flow decisions , action conditions and notify the user groups actions. These files are commonly defined as the part of yacceleratorfulfilmentprocess extension.
​
​
1) Workflow sequence definitions in the form of the XML:
<?xml version="1.0" encoding="utf-8"?>
<process xmlns="http://www.hybris.de/xsd/processdefinition" name="Example" start="Action1">
<action id="Action1" bean="Action1">
<transition name="OK" to="Action2"/>
<transition name="NOK" to="Action3"/>
</action>
<action id="Action2" bean="Action2">
<transition name="OK" to="Action4"/>
</action>
<action id="Action3" bean="Action3">
<transition name="OK" to="Action4"/>
</action>
<action id="Action4" bean="Action4">
<transition name="OK" to="success"/>
</action>
<end id="success" state="SUCCEEDED">Everything was fine</end>
</process>
​
​
Process Definition
​
Process definition defines the set of nodes which are interconnected to each other through ids. We can create the process and execute the process by following code.
​
-
businessProcessService.startProcess(id, processName);
-
businessProcessService.createProcess(id, processName);
​
1) Process Definition: We have to link the process xml file to the ProcessDefinitionResource file.e
<bean id="checkorderProcessDefinitionResource"class="de.hybris.platform.processengine.definition.ProcessDefinitionResource">
<property name="resource"value="classpath:/processdemo/checkorder.xml"/>
</bean>
2)The Actions: We can define the action part of process in the following way.
<bean id="checkOrder"class="de.hybris.platform.fulfilment.actions.CheckOrder" parent="abstractAction">
<property name="checkOrderService"ref="checkOrderService"/>
</bean>
​
3) The Root Tag and Process: The process definition has root tag which contains the name, process start bean and process class.
<?xml version="1.0"encoding="utf-8"?> <process xmlns="http://www.hybris.de/xsd/processdefinition" name="consignmentFulfilmentCustomProcess" start="waitBeforeTransaction" onError="onError" processClass="de.hybris.platform.fulfilment.model.ConsignmentProcessModel">
... ...
</process>
​
4) Node Types: A process has set of nodes which has represent a step in given process. Each node should be defined in the workflow in order of execution of the process. The process has different type of nodes as defined as below.
-
Action Node: Action nodes are the important node of the process they contains the business logic and return type on which basis the next step of the process is executed.
<action id="customProcessCompleted "bean="customProcessCompleted">
<transition name="OK"to="sendOrderCompletedNotification"/>
<transition name="NOK"to="waitForWarehouseSubprocessEnd"/>
</action>​
​
-
Wait Node: This node is used for waiting during the external process get completed. This node will wait for the external process to finish and external process results.
​​
<wait id="waitForWarehouseSubprocessEnd" then="isProcessCompleted" prependProcessCode="false"> <event>${process.code}_ConsignmentSubprocessEnd</event>
</wait>​
-
Notify End: This node is used to perform the notify the user group or user the current state of process.
​
<notify id="notifyadmingroup"then="split">
<userGroup name="admingroup"message="Perform action"/>
<userGroup name="othergroup"message="other message"/>
</notify>​
-
End node: This node is end node of the process and it store the final output of the process.
<end id="error" state="ERROR">All went wrong.</end>
<end id="success" state="SUCCEEDED">Everything was fine</end>​
​
​
​
​
​
Creating a Sample Business Process
​
We have the requirement that after place order we will execute the business process which will send the email notification to Customer and then B2BUnit Partners. We are not going to perform any payment, fraud or consignment related process. Once the email notification is sent the order will be pulled by third party system from hybris for fulfillment.
​
​
​
Step 1: Create a placeOrder-notification-process.xml file.
<process xmlns="http://www.hybris.de/xsd/processdefinition" start="checkOrder" name="placeOrderNotification" processClass="de.hybris.platform.orderprocessing.model.OrderProcessModel">
<action id="checkOrder" bean="checkOrderAction">
<transition name="OK" to="customerOrderPlaceNotification"/>
<transition name="NOK" to="error"/>
</action>
<action id="customerOrderPlaceNotification" bean="customerOrderPlaceNotificationAction">
<transition name="OK" to="partnerOrderPlaceNotification"/>
<transition name="NOK" to="error"/>
</action>
<action id="partnerOrderPlaceNotification" bean="partnerOrderPlaceNotificationAction">
<transition name="OK" to="success"/>
<transition name="NOK" to="error"/>
</action>
<end id="error" state="ERROR">All went wrong.</end>
<end id="failed" state="FAILED">Test Business Process Failed.</end>
<end id="success" state="SUCCEEDED">Test Business Process Successful.</end>
</process>
​
Step 2: Register the process and action beans in the in trainingfulfillmentprocess-spring.xml.
-
Registering the process:
<bean id="placeOrderNotificationProcess" class="de.hybris.platform.processengine.definition.ProcessDefinitionResource" >
<property name="resource" value="classpath:/trainingfulfilmentprocess/process/placeOrder-notification-process.xml"/>
</bean>
​
-
Register the action beans:
<bean id="checkOrderAction" class="com.training.fulfilmentprocess.actions.CheckOrderAction" parent="abstractAction"/>
<bean id="customerOrderPlaceNotificationAction" class="com.training.fulfilmentprocess.actions.CustomerOrderPlaceNotificationAction" parent="abstractAction"/>
<bean id="partnerOrderPlaceNotificationAction" class="com.training.fulfilmentprocess.actions.PartnerOrderPlaceNotificationAction" parent="abstractAction"/>
​
Step 3: Implement all the action classes defined in the previous step. I have given a sample blueprint as below.
-
package com.training.fulfilmentprocess.actions;
-
import de.hybris.platform.orderprocessing.model.OrderProcessModel;
-
import de.hybris.platform.processengine.action.AbstractSimpleDecisionAction;
-
import de.hybris.platform.task.RetryLaterException; import org.apache.log4j.Logger;
-
public class CheckOrderAction extends AbstractSimpleDecisionAction<OrderProcessModel>
-
{
-
private static final Logger LOG = Logger.getLogger(FirstAction.class);
-
@Override public Transition executeAction(final OrderProcessModel var1) throws RetryLaterException, Exception
-
{
-
// XXX Auto-generated method stub
-
LOG.info("CheckOrderAction!!!");
-
return Transition.OK;
-
}
-
}
Step 4: Start the business process by following service as per the requirement. In above scenario we can place this code in place order flow.
-
Create the Business process instance.
final OrderProcessModel placeOrderNotificationProcess = (OrderProcessModel) getBusinessProcessService() .createProcess("placeOrderNotificationProcess" + System.currentTimeMillis(), "placeOrderNotificationProcess");
​
-
Start the Business Process.
getBusinessProcessService().startProcess(placeOrderNotificationProcess);​
​
​
​
Business Process Action Synchronous Way
We can run Action nodes in a business process definition asynchronously or synchronously. In the asynchronous mode, an action node is treated as a single and separate task when run by the task engine. In the synchronous mode, an action node is run in the same task as the preceding node.We
By default actions are run in asynchronous manner, we can explicitly make them synchronous by adding the following property in local.property file : processengine.process.canjoinpreviousnode.default=false
​
​
​
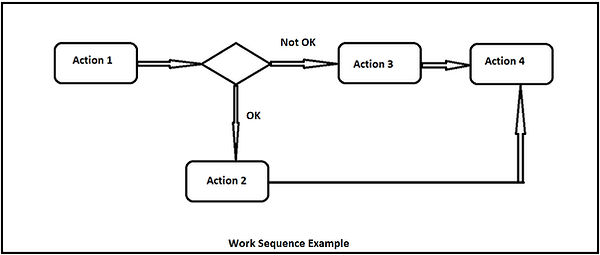
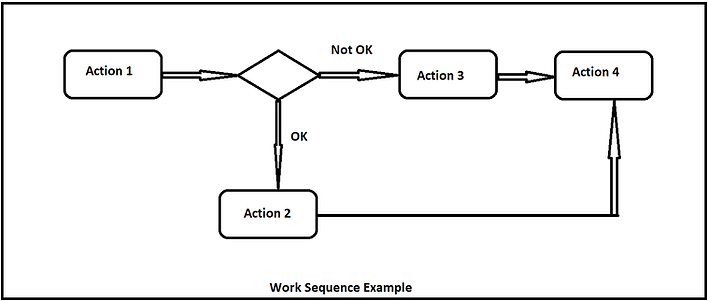
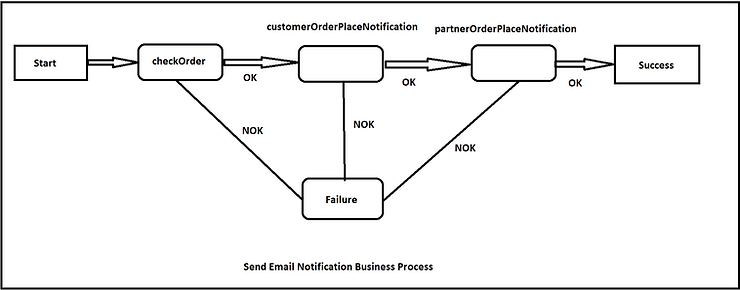